문제
풀이
#include <string>
#include <vector>
#include<cstdlib> //abs for int, long int
#include <cmath>
using namespace std;
int cal(vector<int> po_1, vector<int> po_2)
{
return abs(po_1[0] - po_2[0]) + abs(po_1[1] - po_2[1]);
}
string solution(vector<int> numbers, string hand) {
string answer = "";
vector<int> lh = {0,3}; // x,y
vector<int> rh = {2,3};
vector<int> n_po = {0,0};
char h = hand == "left" ? 'L' : 'R';
for(int i=0; i<numbers.size(); i++){
int num = numbers[i];
if(num == 0) n_po = {1,3};
else n_po = {(num-1)%3, (num-1)/3};
if(num == 1 || num == 4 || num == 7){
answer += 'L';
lh = n_po;
}
else if(num == 3 || num == 6 || num == 9){
answer += 'R';
rh = n_po;
}
else { //2 5 8 0
if(cal(lh,n_po) < cal(rh,n_po)){
answer += 'L';
lh = n_po;
}
else if(cal(lh,n_po) > cal(rh,n_po)){
answer += 'R';
rh = n_po;
}
else {
h == 'L' ? answer += 'L' : answer += 'R';
h == 'L' ? lh = n_po : rh = n_po;
}
}
}
return answer;
}
해결 과정
1. 숫자 키패드의 위치를 좌표화 한다.
2. 입력할 숫자(num)가 2,5,8,0일 경우 왼손 위치(lh) 오른손 위치(rh)의 거리를 구하고, 더 가까운 쪽 손을 선택한다.
3. 각각 구한 거리가 같을 경우 hand로 넘어온 문자를 이용해 왼손잡이, 오른손잡이를 구분한다.
처음엔 두 좌표 간 거리를 구하는 방법을 사용하려 했으나, 한 손이 7 한 손이 0 일 경우 0일 때의 거리가 더 길게 나오기 때문에 오류가 발생했다. 따라서 두 좌표 간의 이동거리를 구해 해결했다.
다른 사람 풀이
#include <string>
#include <vector>
#include <math.h>
using namespace std;
string solution(vector<int> numbers, string hand) {
string answer = "";
int curr_L = 10;
int curr_R = 12;
for(int i = 0; i < numbers.size(); i++) {
if(numbers[i] % 3 == 1) {
answer += "L";
curr_L = numbers[i];
}
else if(numbers[i] % 3 == 0 && numbers[i] != 0) {
answer += "R";
curr_R = numbers[i];
}
else {
int tmp = numbers[i];
if(numbers[i] == 0) tmp = 11;
int dist_L, dist_R;
if(curr_L % 3 == 1) dist_L = abs(tmp - curr_L - 1) / 3 + 1;
else dist_L = abs(tmp - curr_L) / 3;
if(curr_R % 3 == 0) dist_R= abs(tmp - curr_R + 1) / 3 + 1;
else dist_R = abs(tmp - curr_R) / 3;
if(dist_L == dist_R) {
if(hand[0] == 'l') {
answer += "L";
curr_L = tmp;
}
else {
answer += "R";
curr_R = tmp;
}
}
else {
if(dist_L < dist_R) {
answer += "L";
curr_L = tmp;
}
else {
answer += "R";
curr_R = tmp;
}
}
}
}
return answer;
}
벡터를 사용해 좌표처럼 사용하거나, 따로 함수를 생성하지 않고도 각 키패드 간의 거리를 구할 수 있었다...!
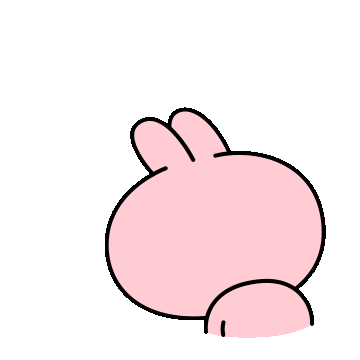
https://blockdmask.tistory.com/70
[C++] vector container 정리 및 사용법
안녕하세요. BlockDMask 입니다. 오늘은 C++ STL의 sequence container 중에 정말 자주 쓰는 vector에 대해서 알아보겠습니다. <목차> 1) vector container 란? 2) vector의 사용 3) vector의 생성자와 연산..
blockdmask.tistory.com
조건 연산자:?:
자세한 정보: 조건 연산자:?:
docs.microsoft.com
https://luv-n-interest.tistory.com/975
C++ 에서의 자료구조 Array 대신 Vector를 쓰는 이유
C++에서는array도 제공하지만 vector도 제공한다. 왜 우리는 vector를 쓰는가? 우선 array는 컴파일할 때 크기가 결정된다. 즉, 미리 알고 선언해야한다는 말이다. 크기가 고정되어있으므로 원소의 데이
luv-n-interest.tistory.com
'Algorithm > 카카오기출' 카테고리의 다른 글
[프로그래머스]비밀지도 (0) | 2022.01.15 |
---|---|
[프로그래머스] 실패율 (0) | 2022.01.14 |
[프로그래머스]크레인 인형뽑기 게임 (0) | 2022.01.12 |
[프로그래머스]숫자 문자열과 영단어 (0) | 2022.01.08 |
[프로그래머스]신규 아이디 추천 (0) | 2022.01.05 |